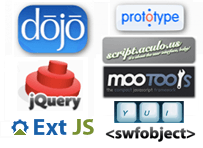
I don’t use a lot of JavaScript, but as you probably know, I’m a fan of offloading things to other people when it can save me the hassle of doing it myself.
A CDN — short for Content Delivery Network — distributes your static content across servers in various, diverse physical locations. When a user’s browser resolves the URL for these files, their download will automatically target the closest available server in the network.
What are the AJAX APIs?
As you may have heard, since May 27, 2008 Google has released a new client-side API. It permits users to download some popular JavaScript libraries directly from its servers, including Prototype, jQuery, Scriptaculous, MooTools, and Dojo. In addition, the API will serve the requested files compressed by default (even though this feature can be disabled via client-side scripting), in this manner decreasing their respective download times.
Google’s AJAX APIs let you implement rich, dynamic web sites entirely in JavaScript and HTML. You can add a map to your site, a dynamic search box, or download feeds with just a few lines of JavaScript.
If you want to play around with them, try using the Interactive Code Playground. Plus, the Google API Libraries for Google Web Toolkit has bindings for the Maps, Search, Visualization, and Language APIs.
Why you should let Google host Javascript libraries
- Decreased Latency: In the case of Google’s AJAX Libraries CDN, what this means is that any users not physically near your server will be able to download JavaScript library faster than if you force them to download it from your arbitrarily located server.
There are a handful of CDN services comparable to Google’s, but it’s hard to beat the price of free! This benefit alone could decide the issue, but there’s even more. - Increased parallelism: To avoid needlessly overloading servers, browsers limit the number of connections that can be made simultaneously. Depending on which browser, this limit may be as low as two connections per hostname.
Using the Google AJAX Libraries CDN eliminates one request to your site, allowing more of your local content to downloaded in parallel. It doesn’t make a gigantic difference for users with a six concurrent connection browser, but for those still running a browser that only allows two -
[adsense ad_client=ca-pub-0297902515090400 ad_slot=3999285131 width=336 height=280]
Better caching: Potentially the greatest (yet least mentioned) benefit of using the Google AJAX Libraries CDN is that your users may not need to download jQuery at all.
No matter how aggressive your caching, if you’re hosting jQuery locally then your users must download it at least once. A user may very well have dozens of identical copies of jQuery-1.3.2.min.js in their browser’s cache, but those duplicate files will be ignored when they visit your site for the first time.
On the other hand, when a browser sees multiple subsequent requests for the same Google hosted version of jQuery, it understands that these requests are for the same file. Not only will Google’s servers return a 304 “Not Modified” response if the file is requested again, but also instructs the browser to cache the file for up to one year.
This means that even if someone visits hundreds of sites using the same Google hosted version of jQuery, they will only have to download it once. - High performance: HTTP compression minimizes the size of the download, minimized versions of the each library are available to further reduce download size and you can specify which version of a library should be used with a hard-coded URL or allow for automatic version upgrades using the google.load(); function
Which Libraries are Supported?
The current version of the plugin supports the following JavaScript libraries:
- jQuery
- jQuery UI
- Prototype
- Scriptaculous
- MooTools
- Dojo
- SWFObject
- Yahoo! User Interface Library (YUI)
- Ext CoreNew!
- Chrome FrameNew!
Using google.load()
The most powerful way to load the libraries is by using google.load() to name a library and your prefered version. E.g.:
google.load("jquery", "1.4.0"
google.load("jqueryui", "1.7.2"
google.load("prototype", "1.6.1.0"
google.load("scriptaculous", "1.8.3"
google.load("mootools", "1.2.4"
google.load("dojo", "1.4.0"
google.load("swfobject", "2.2"
google.load("yui", "2.8.0r4"
google.load("ext-core", "3.1.0"
As you can see in the code snippets above, the first argument to google.load
is the name of a library. The second argument is a version specification. The complete list of Ajax libraries is a growing collection of the most popular, open source JavaScript libraries.
The versioning system allows your application to specify a desired version with as much precision as it needs. By dropping version fields, you end up wild carding a field.
I must say, though, I’m not a fan of the google.load() method, even though that’s what Google recommends.
<script type="text/javascript" src="http://www.google.com/jsapi"></script>
<script type="text/javascript">
// You may specify partial version numbers, such as "1" or "1.3",
// with the same result. Doing so will automatically load the
// latest version matching that partial revision pattern
// (e.g. 1.3 would load 1.3.2 today and 1 would load 1.4).
google.load("jquery", "1.4"
google.setOnLoadCallback(function() {
// Place init code here instead of $(document).ready()
});
</script>
Doing that removes the reliability of things like $(document).ready(), because you’d have to wait for the load() function to finish, well, loading the library. There’s a way around that by using the google.setOnLoadCallback() and passing it a function, but eh. I just don’t like it.
The Right Way to including AJAX Libraries API in WordPress
After reading several posts on the subject, listed at end of post, I came up with the greatest way of calling jQuery into WordPress (you can do the same with other javascript libraries). Here is what the following code will do for you.
- Deregister the local copy of Jquery used by wordpress
- Call Jquery hosted from Google
- Register Jquery from Google and place it in the footer
- Register your other external js files and place them after Jquery
<?php
// Footer scripts
function jquery_init() {
if (!is_admin()) {//load scripts for non admin pages
wp_deregister_script('jquery');//deregister current jquery
wp_register_script('jquery', 'http://ajax.googleapis.com/ajax/libs/jquery/1.3.2/jquery.min.js', false, '1.3.2', true);//load jquery from google api, and place in footer
wp_enqueue_script('jquery');
// load a additional js files
wp_enqueue_script('my_script', get_bloginfo('template_url') . '/js/my-script.js', array('jquery'), '1.0', true);
wp_enqueue_script('my_script2', get_bloginfo('template_url') . '/js/my-script2.js', array('jquery'), '1.0', true);
}elseif (is_admin()){//load scripts for admin page
wp_enqueue_script('my_admin_script', get_bloginfo('template_url') . '/js/my-admin-script.js', array('jquery'), '1.0', true);
wp_enqueue_script('my_admin_script2', get_bloginfo('template_url') . '/js/my-admin-script2.js', array('jquery'), '1.0', true);
}
}
add_action('init', 'jquery_init');
// END :Footer scripts
?>
Ok, there are couple of things I want to go over here.
- You should always put all of your scripts in the footer. Why? Because, when a web page loads the markup it loads from top to bottom. So, if you have several scripts at the top of the page your users have to wait for all that stuff to load in the background before any of the lower content will load. That’s almost as unbearable as Steve Austin running in slow-mo. Also, most of your javascript is no good unless it has content to play with, so just put the scripts at the bottom of the page and it will load faster with every thing working correctly. If you don’t believe me just view the source on my website and see, all the scripts in the footer.
- Have WordPress place your external scripts according to their javascript library. The wp_engueue_ascript() function allows for an argument called $deps, which you can use to attach any other external scripts to its library and then WordPress will place it on the page accordingly.
Conclusion
What do you think? Are you using the Google AJAX Libraries CDN on your sites? Can you think of a scenario where the google.load()
method would perform better than simple <script> declaration?
Do you know?: Microsoft now provides a similar service for MicrosoftAjax.js.
Hi Nguyễn,
Nice informative post! I’ve learnt a few things here, especially the part about always putting all of your scripts in the footer of your markup. I never knew that. Thanks.
Jon
Hope you can use it on your website :)
Hi Nguyen,
Nice post. I’ve recently been looking into converting our website into WordPress and your point about being able to have WordPress place external scripts according to their Javascript library is another argument for doing so.
I’m just a little worried about SEO factors, as I’ve heard conflicting stories.
Anyhow, great post. Keep it up!
Gav.
Yay, you finally are more thorough than the other 10 sites I’ve read! It’s nice to see someone do their research before copying and pasting some incorrect method. The authors of “Digging into WordPress” confirm your method of including jquery from google cdn. My biggest question. Scriptaculous and Prototype. They are libraries included in wordpress, but I’ve never seen someone access them from the google CDN. They aren’t included in jQuery, so why isn’t anyone suggesting that as well? Or is it assumed as another of my scripts? I’ve just never seen anyone specify it.
Thanks!
One more question.
When does one put functions, scripts, includes, etc. in the functions.php file or in the header/footer/or template itself?
Example, I’m using a premium theme, (ElegantThemes) and so far everything they do is superb. But like everyone else I’ve seen, they load their custom scripts in the header files. As far as I’m concerned, if if it’s only for posts & pages, does it make any difference?
For that matter, does it make any difference to call stylesheets from the header or functions.php?
THANKS!
Please, I want to know the particular code I will use to activate visual editor.Visual editor is not working on my wordpress.
Thank you
I guess you don’t active the classic editor because the new Visual Editor will replace it soon in next version of WordPress