Lazy loader is a jQuery plugin written in JavaScript. It delays loading of images in (long) web pages. Images outside of viewport (visible part of web page) wont be loaded before user scrolls to them. Using lazy load on long web pages containing many large images makes the page load faster. Browser will be in ready state after loading visible images. In some cases it can also help to reduce server load. Lazyloader inspired by YUI ImageLoaderUtility by Matt Mlinac.
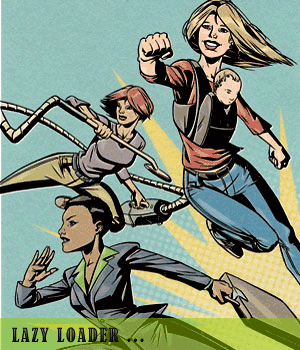
Lazy loading is a design pattern commonly used in computer programming to defer initialization of an object until the point at which it needed. It can give to efficiency in the program’s operation if properly and appropriately used.
How the logic works?
Using JavaScript, it is possible to detect the following:
- the coördinates of the part of the page (x-y) we’re viewing
- the coördinates of a specific element
- the width-height of the viewport
Having these inputs, we can easily understand if a given element is in the viewpoint or not. And, after it becomes “in”, we can make a JavaScript call to load the content.
How to use?
Include them in your header:
<script src="jquery.js" type="text/javascript"></script>
<script src="jquery.lazyload.js" type="text/javascript"></script>
and in your code do:
$("img").lazyload();
This causes all images below the fold to be lazy loaded.
Setting sensitivity
There are options for control maniacs who need to finetune. You can set threshold on how close to the edge (don’t push me too far) image should come before it is loaded. Default is 0 (when it is visible).
$("img").lazyload({ threshold : 200 });
Setting threshold to 200 causes image to load 200 pixels before it is visible.
Placeholder image
You can also set placeholder image and custom event to trigger loading. Place holder should be a url to image. Transparent, grey and white 1×1 pixel images are provided with plugin.
$("img").lazyload({placeholder : "img/grey.gif"});
Event to trigger loading
Event can be any jQuery event such as click or mouse over. You can also use your own custom events such as sporty or foobar. Default is to wait until user scrolls down and image appears on the window. To prevent all images to load until their grey placeholder image is clicked you could do:
$("img").lazyload({
placeholder : "img/grey.gif",
event : "click"
});
Using effects
By default plugin waits for image to fully load and calls show to show it. You can use any effect you want. Following code uses fadeIn effect. Check how it works at effect demo page.
$("img").lazyload({
placeholder : "img/grey.gif",
effect : "fadeIn"
});
Images inside container
You can also use plugin for images inside scrolling container, such as div with scroll bar. Just pass the container as jQuery object. There is a demo for horizontal and vertical container.
#container {
height: 600px;
overflow: scroll;
}
$("img").lazyload({
placeholder : "img/grey.gif",
container: $("#container")
});
When images are not sequential
After scrolling page Lazy Load loops though unloaded images. In loop it checks if image has become visible. By default loop is stopped when first image below the fold (not visible) is found. This is based on following assumption. Order of images on page is same as order of images in HTML code. With some layouts assumption this might be wrong. You can control loading behaviour with failurelimit option.
$("img").lazyload({
failurelimit : 10
});
Setting failurelimit to 10 causes plugin to stop searching for images to load after finding 10 images below the fold. If you have a funky layout set this number to something high.
Delayed loading of images.
Not exactly feature of Lazy Load but it is also possible to delay loading of images. Following code waits for page to finish loading (not only HTML but also any visible images). Five seconds after page is finished, below the fold images are loaded automatically. You can also check the delayed loading demo.
$(function() {
$("img:below-the-fold").lazyload({
placeholder : "img/grey.gif",
event : "sporty"
});
});
$(window).bind("load", function() {
var timeout = setTimeout(function() {$("img").trigger("sporty")}, 5000);
});
Known problems
Due to WebKit bug #6656 Lazy Loading wont give you any improvements in Safari. It will load all images you wanted it or not. You can workaround this with
Also you are using Mint you should have mint tag in the header of the page. Mint tag in the end of the page interferes with Lazy Load plugin. This is rather peculiar problem. If somebody finds an answer let me know.
Lazy Loader Homepage (jQuery plugins)
Get jQuery Lazy Loader Minified (JS, 2.92KB)
Another Javascript Libraries Images Lazy Loading Solutions
- MooTools LazyLoad is a customizable MooTools plugin that allows you to only load images when the user scrolls down near them.
- YUI 3 – ImageLoader Utility allows you as an implementor to delay the loading of images on your web page until such a time as your user is likely to see them. This can improve your overall page load performance by deferring the loading of some images that are not immediately visible at the time the page first renders. Because images are often the heaviest components of a given page, deferring the loading of some images can yield a marked improvement in the way the page “feels” to your user.
- Prototype – lazierLoad lazierLoad automatically hooks itself to the page, finds all images and only loads those appearing “above the fold” resulting in faster page loads. The images not located in the viewport, are not loaded until they appear within it (viz. when the user scrolls down).
There is already a WordPress plugin doing the same task as lazy loader, we don’t need to do complicated things above anymore. :)
There is already a WordPress plugin doing the same task as lazy loader, we don’t need to do complicated things above anymore.
This helps me a lot when loading images it saves the bandwidth as well as the scripts on ie runs smoothly just like on my site
Great tutorial! thank you.
WP plugin??? who cares. many, many, many more sites out there that benefit from this. when you say “we don’t need” don’t include me in there. WP is such a small fry.