As you know, we’re living in Mobile era as know as Mobilegeddon. So, the design trends has changed then focusing on mobile devices as well as it.
What’s about Flat & Material Design?
Flat design is a minimalistic design approach that emphasizes usability. As the name indicates, flat design is defined by flatness of style: simplifying an interface by removing extra elements such as shadows, bevels, textures and gradients that create a 3D look. Everything is clear and understandable, and the design relies mostly on colours and icons to give meaning.
New websites are all flat so will be new email templates as well.
In today’s post we have gathered trendy flat style responsive email templates to market your online business.
1. Flattie – $19
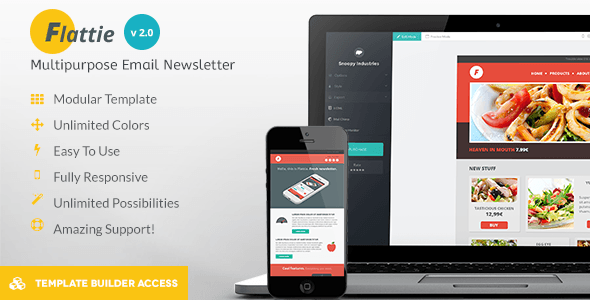
Flattie is an email built for companies, enterprises, businesses or freelancers who want to find a beautiful and clean solution to their email marketing needs. It’s Mailchimp and Campaign Monitor ready, and also built with both Responsive and Non-Responsive templates, so you can choose what suits your company best and promote with a very beautiful looking template which is certain to attract the attention of your customers and inquire their curiosity.
2. Titan – Responsive Email + Themebuilder Access – $19
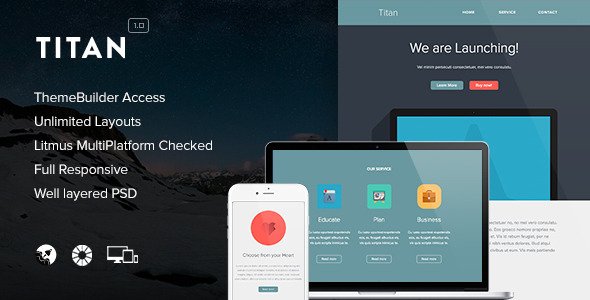
Features
- A link to our ThemeBuilder
- Export to desktop
- Change background image / patterns
- Drag & Drop modules
- Duplicate or hide modules
- Unlimited Structures and Colours
- Full Width Design
- Clean Commented Code
- PSD Photoshop File included
- Documentation
Email Services Compatibility
- MailChimp
- Icontact & Campaign Monitor
- Tested with Litmus
- Mobile responsive
- And many more!
3. Flattro – $18
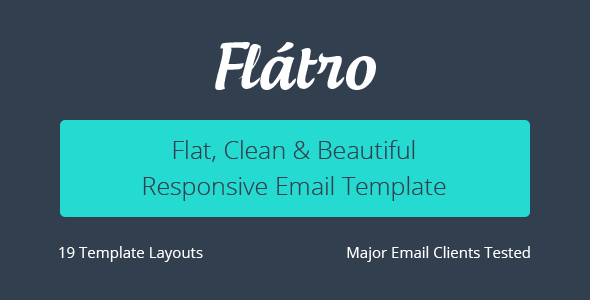
Flatro – A multipurpose corporate look email newsletter. It is simple, clean and trendy. The responsiveness makes you easy to target all kind of audience and displays better in any device. Testing in MailChimp, Campaign Monitor and Thunderbird ensure that the templates are free from any display bug.
Features:
- Total 19 email newsletter templates
- Maximum of three columns
- 9 basic layouts and 9 main layouts, ready to instant use
- Major mail clients and browser display tested
- Gmail, Yahoo, Rediffmail, Outlook, Thunderbird, Opera mail and more
- Firefox, Chrome, IE and Opera
- Well organized and layered PSD files
- Easy understanding commented code
- 16 modules
- Help document
- Post sales support
4. Flick – $18
Flick a minimalist email template design come with Flat UI style. It’s came with 2 styles: Boxed and Letter like layout style, also include slide icons ready to use.
- Template Builder by Campaign Monitor , MailChimp , StampReady , MyMail
- Compatible with FreshMail
- 6 color scheme (Blue, Cyan, Green, Purple, Pink, Red).
- Commented HTML.
- Flexible table structure (delete/copy/replace).
- Responsive for your mobile device..
- Try test sending directly at the demo page
- Compatible with StampReady builder
- 15 Reapeatable Modules.
- Advanced lossy compression reduce png,jpg file size more than 70%.
- Compatible with awesome WordPress plunin MyMail
- Sliced Icons ready to use (from Designmodo)
5. ONETOUCH – $16
ONE-TOUCH is designed with 05 great looking layout’s. It have 02 color combination. Total 10HTML along with 10 layered PSD. This will help you to promote your professional email marketing worldwide.
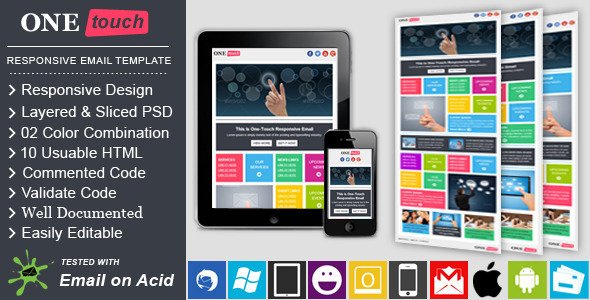
ONE-TOUCH Specification’s and features:
• Responsive Design.
• 10 HTML’s.
• 05 Layout’s.
• 10 PSD files included.
• 10HTML Files fully customizable and ready for general use.
• Valid HTML 4 .01 Transitional.
• Inline CSS.
• TABLE Based structure.
• Dreamweaver Validate.
• w3schools validate.
• Unique layout.
• Well commented code.
• All major email clients tested.
• Organized coding structure.
• Easy to customize.
• Well Documented.
• Quick support.
• Test result screenshot attached.
• Tested for all major email/desktop/email clients including- Gmail, Yahoo, Hotmail, Outlook 2007/2010, Android, iPad, Iphone etc.
• Renders perfectly on all web browsers.
6. FlatroWay – Metro Flat Responsive Email Template – $18
Flatroway is an elegant mix of Metro And Flat design trends, a powerful responsive email package, including both DARK and LIGHT versions. Responsive to your needs, handcrafted carefully for you, tested thoroughly over several weeks on more than 40 email clients , including the most popular IOS APP Mailbox and Outlook Mobile APP.
7. Ballio – Flat Responsive Email With Template Builder – $19
BALLIO – Flat Style and Multi Usage Responsive Email Template is awesome and mordern template which comes in 24 color themes,10 layouts each, total 240 template pages. With Template Builder V2.1
How the fuck I can download it for free if It redirects me to the Purchase page?
There’re free at the time when the article was published.